Schedule Service Restarts with PowerShell
Ben Liebowitz
- 0
- 3189
Have you ever had a service randomly stop on you over and over again?! In my homelab environment, I use a company called Itarian to patch my servers, control them remotely, etc. One thing I found is that the main Itarian service, ITSMService, keeps crashing! You can see below, a few of the servers are grey.

I wrote a quick little PowerShell script to check if the service is running and if not, start it!
##############################################
#
# Powershell script to check ITSMSerivce
# Written by BLiebowitz on 11/29/23
#
##############################################
# assign variable to get the service
$itsm = get-service -name "ITSMService"
# Run IF statement to check if the ITSMService is stopped
if ($itsm.status -like "stopped") {
# Since the service is stopped, start the service
$itsm | Start-Service
} else {
# Do nothing, the service is already running
}
Next, I used Task Scheduler to run the script on a regular basis.
BUT FIRST, you need to make sure to set the execution policy to allow you to run unsigned scripts. Since this is a lab, I set mine to bypass, but you can set yours accordingly. Here are the descriptions from https://learn.microsoft.com/en-us/powershell/module/microsoft.powershell.security/set-executionpolicy?view=powershell-7.4.
- AllSigned. Requires that all scripts and configuration files are signed by a trusted publisher, including scripts written on the local computer.
- Bypass. Nothing is blocked and there are no warnings or prompts.
- Default. Sets the default execution policy. Restricted for Windows clients or RemoteSigned for Windows servers.
- RemoteSigned. Requires that all scripts and configuration files downloaded from the Internet are signed by a trusted publisher. The default execution policy for Windows server computers.
- Restricted. Doesn’t load configuration files or run scripts. The default execution policy for Windows client computers.
- Undefined. No execution policy is set for the scope. Removes an assigned execution policy from a scope that is not set by a Group Policy. If the execution policy in all scopes is Undefined, the effective execution policy is Restricted.
- Unrestricted. Beginning in PowerShell 6.0, this is the default execution policy for non-Windows computers and can’t be changed. Loads all configuration files and runs all scripts. If you run an unsigned script that was downloaded from the internet, you’re prompted for permission before it runs.
Back to Task Scheduler. Click ACTION – CREATE A BASIC TASK. Give it a name and click NEXT.

Choose DAILY and click next. (I want it to run hourly, but that isn’t an option here. See at the end how do achieve hourly.)
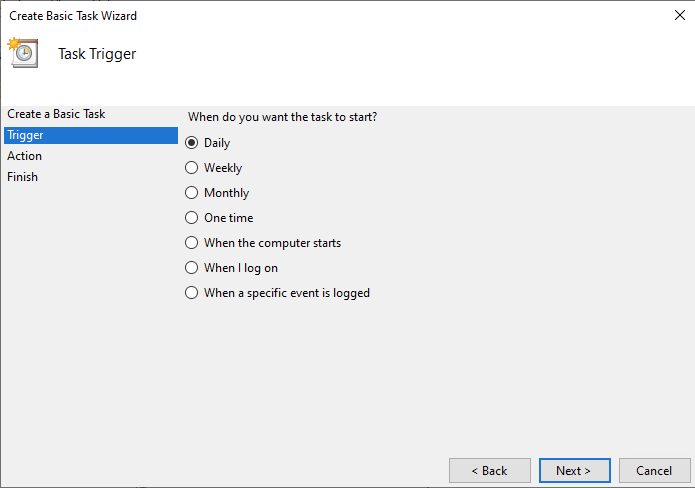
Choose a start time and click NEXT.

We want to start a program. Click NEXT.

The program is PowerShell.exe, under add arguments, put: -file <path to .ps1>
Click NEXT

Click the checkbox to open the properties dialog box and click FINISH.

First, click the RUN WITH HIGHEST PRIVILEGES Checkbox. and RUN whether user is logged in or not. Then click the Triggers tab.

Select DAILY and click EDIT.

Click the REPEAT TASK EVERY checkbox. Make sure it’s set to 1 hour and change the duration drop-down to INDEFINITELY. Click OK twice.
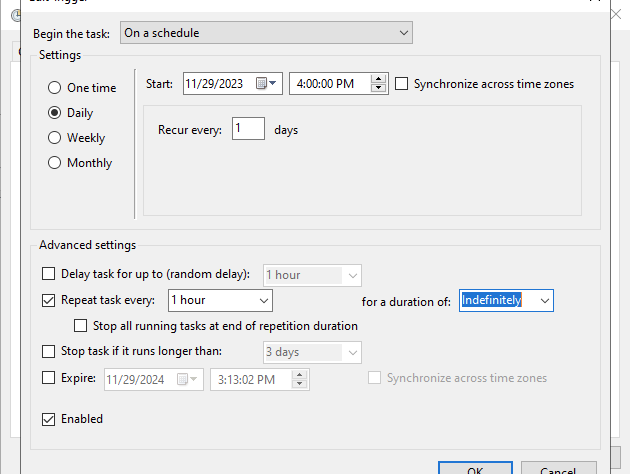
Next, you’ll be prompted to store the password for the user that created the task.
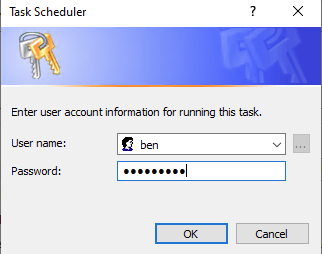
However, this is a PowerShell post, so lets automate creating the scheduled task too!
# Assigns the action or the task being scheduled.
$action = New-ScheduledTaskAction -execute "PowerShell.exe" -argument "-file c:\ps1\itsmservice.ps1"
# A Trigger can be anything from on a schedule (as we have here), at startup, log on, etc.
$trigger = New-ScheduledTaskTrigger -Once -At (get-date) -RepetitionInterval (New-TimeSpan -Minutes 60)
# Since we're executing a script, this variable is the path to said script.
$taskpath = "c:\ps1\itsmservice.ps1"
# Name the task.
$TaskName = "ITSMService"
# I like to describe what the task does here
$Description = "Scheduled Task to Restart The ITSM Service if stopped"
# Create the scheduled task!
Register-ScheduledTask -Action $action -Trigger $Trigger -TaskName $Taskname -Description $Description
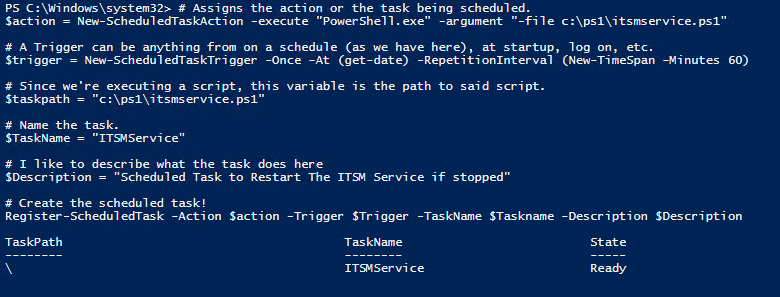
Now, your service will automatically be restarted on the hour, every hour (if needed)!
Ben Liebowitz, VCP, vExpert
NJ VMUG Leader